I had a requirement to write a webservice in windows server 2008 with IIS 7. So this is just a summary of the things we did and the troubles we came across,
Step - 1 : Writing the code behind
DBService.asmx.cs
[WebMethod]
public DataSet getOrderDetails(string OrderNumber)
{
OracleConnection con = null;
OracleCommand cmd = null;
string query
= String.Empty;
query = "Select * from table_name where CUSTOMER_PO = '" +
OrderNumber + "' ORDER BY REQUEST_DATE ASC ";
try
{
con = new
OleDbConnection(System.Configuration.ConfigurationManager.AppSettings["connstring"].ToString());
con.Open();
cmd = new OracleCommand(query, con);
DataSet orderDataSet =
new DataSet();
OracleDataAdapter adapter = new OracleDataAdapter(cmd);
adapter.Fill(orderDataSet);
con.Close();
return orderDataSet;
}
catch (Exception e)
{
query = e.Message;
throw;
}
web.config

Step -2: Deploying
1. Publish the webservice.
2. Create a virtual directory in IIS
3. Bind the published webservice path to the virtual directory created.
4. In IIS 7, individual app pool is created for every virtual directory.
5. Browse the application link(find in the right side) to view your application.
Possible troubles/errors/exceptions
1. If we are not able to view the webmethods writen, Possibly the directory browsing is not enabled. We can enable it. It is quite simple.
Solution:
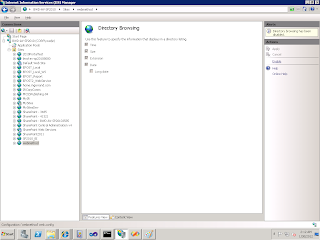
2. If the same webservice is accessed from different server, We may not get the input box to enter the user data. In this case web.config should be modified to include this block,
web.config
3. TNS entry error- This is a very often error which can be solved by just having an entry for the datasource, in the TNS file which can be found somewhere similar to C:\Orawin\network\admin
It happened to me like, my code was working perfect with all the servers except with the development environment.
Later we found that the dev server was 64 bit which caused the problem. So we made changes like building the code in X64 configuration/ enabling or disabling 32 bit in IIS. We replaced Oledb with Oracle connection and replaced the conn string with the actual TNS entry. Finally having the reference "using Oracle.DataAccess.Client;" and modifying the connection object like
"con = new OracleConnection("Actual TNS entry");" and it worked.